https://www.acmicpc.net/problem/10845
10845번: 큐
첫째 줄에 주어지는 명령의 수 N (1 ≤ N ≤ 10,000)이 주어진다. 둘째 줄부터 N개의 줄에는 명령이 하나씩 주어진다. 주어지는 정수는 1보다 크거나 같고, 100,000보다 작거나 같다. 문제에 나와있지
www.acmicpc.net
문제 분석
선형 자료구조인 큐(Queue)를 구현하는 문제이다.
큐는 데이터가 가장 마지막(위쪽)으로 추가되며, 가장 처음 들어왔던 데이터가 먼저 나가게 되는 선입선출(First in First Out)구조다.
풀이
클래스 내 필드로는 int 배열인 queue, 큐의 사이즈를 담고 있는 size 를 두었다.
각 메소드 별 설명은 다음과 같다.
- push()
삽입 연산으로, 마지막 위치에 추가해야 하기 때문에 size를 1추가 후
새로운 사이즈로 배열을 생성하여 기존 배열에 담긴 요소들을 복사해 넣고 마지막 인덱스에 새로운 값 추가 - pop()
삭제 연산으로, 첫번째 요소를 삭제해야 한다. size를 1뺀 후
새로운 사이즈로 배열을 생성하여 기존 배열의 요소들을 인덱스 1번부터 마지막 인덱스까지 복사 - size()
size 필드값 출력 - empty()
size 값을 확인하여 0이라면 true, 아니라면 false 출력 - front()
0번째 인덱스 값 출력 - back()
마지막 인덱스 값 출력
코드
public static void main(String[] args) throws Exception{
BufferedReader bf = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(bf.readLine());
Queue queue = new Queue();
for (int i = 0; i < n; i++) {
String command = bf.readLine();
String[] arr = command.split(" ");
switch(arr[0]) {
case "push": queue.push(Integer.parseInt(arr[1]));break;
case "pop": queue.pop();break;
case "size": queue.size();break;
case "empty": queue.empty();break;
case "front": queue.front();break;
case "back": queue.back();break;
}
}
}
public static class Queue {
private int size;
private int[] queue;
public void push(int x) {
this.size++;
int[] newQueue = new int[this.size];
if (this.queue == null) {
newQueue[0] = x;
} else {
newQueue = Arrays.copyOf(this.queue, this.size);
newQueue[newQueue.length-1] = x;
}
this.queue = newQueue;
}
public void pop() {
if (this.size < 1) {
System.out.println(-1);
this.queue = null;
} else {
this.size--;
System.out.println(this.queue[0]);
int[] newQueue = new int[this.size];
newQueue = Arrays.copyOfRange(this.queue, 1, this.size+1);
this.queue = newQueue;
}
}
public void size() {
System.out.println(this.size);
}
public void empty() {
if (this.size == 0) {
System.out.println(1);
} else {
System.out.println(0);
}
}
public void front() {
if (this.size == 0) {
System.out.println(-1);
} else {
System.out.println(this.queue[0]);
}
}
public void back() {
if (this.size == 0) {
System.out.println(-1);
} else {
System.out.println(this.queue[this.size-1]);
}
}
}
댓글 피드백은 언제나 환영합니다!
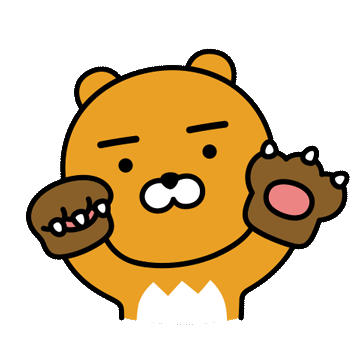
'CodingTest > 백준' 카테고리의 다른 글
백준 1874번 - 스택 수열 (1) | 2023.12.08 |
---|---|
백준 9012번 - 괄호 (0) | 2023.09.28 |
백준 4153번 - 직각삼각형 (0) | 2023.09.22 |
백준 1654번 - 랜선 자르기 (0) | 2023.09.22 |
백준 10828번-스택(JAVA) (0) | 2020.09.13 |